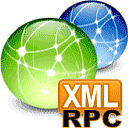
Once I needed to make some XML-RPC method calls, but faced with the lack of simple implementation of it.
I tried to use different libraries, but they are too complicated. For, example PEAR's xml-rpc implementation is quite difficult to install on Windows and not always possible to install on Linux shared hosting. And XML-RPC for PHP at sourceforge have some problems with UTF-8 characters encoding. So I created my on simple XML-RPC client.
The following class will let you perform XML-RPC method calls from PHP. It also solves encoding problems, since standard PHP xml-rpc methods works bad with non-ANSI UTF-8 characters (e.g. Cyrillic)
class XmlRpcClient { public $encoding; public $server; public $endpoint; public function __construct($server, $endpoint, $encoding = 'UTF-8') { $this->server = $server; $this->endpoint = $endpoint; $this->encoding = $encoding; } public function call($method, $params = array()) { $request = xmlrpc_encode_request( $method, $params, array('escaping' => 'markup', 'encoding' => $this->encoding) ); $context = stream_context_create(array('http' => array( 'method' => "POST", 'header' => "Content-Type: text/xml User-Agent: PHPRPC/1.0 Host: ".$this->server." ", 'content' => $request ))); $server = "http://".$this->server.$this->endpoint; $file = file_get_contents($server, false, $context); return xmlrpc_decode($file); } }
PS: this is actually an old post from the old version of my blog and I'm publishing it again for 2 reasons:
- It may be useful for somebody.
- I have a goal to publish 1 blog post per month and since I don't have time to create a new one, I'll publish the old one.
And Happy New Year to everyone. I wish you productivity, self-fullfilment and enough time to implement the best of your ideas right here and right now!